Dictionaries are a fundamental data structure in Python, offering a versatile way to store and manipulate data in a key/value pair format. They are widely used in various applications, from managing configuration settings to representing real-world entities. In this comprehensive blog, we will dive deep into Python dictionaries, focusing on the art of adding key/value pairs. You’ll learn not only the basics but also some powerful dictionary hacks to enhance your Python programming skills.
Embarking on a Journey into Python Dictionaries
Python dictionaries serve as a fundamental and versatile data structure, facilitating efficient management of key-value pairs. In this blog, we embark on a journey into the world of Python dictionaries, where we will explore their inner workings, provide insights, and share some valuable tips and hacks to make the most of these powerful tools.
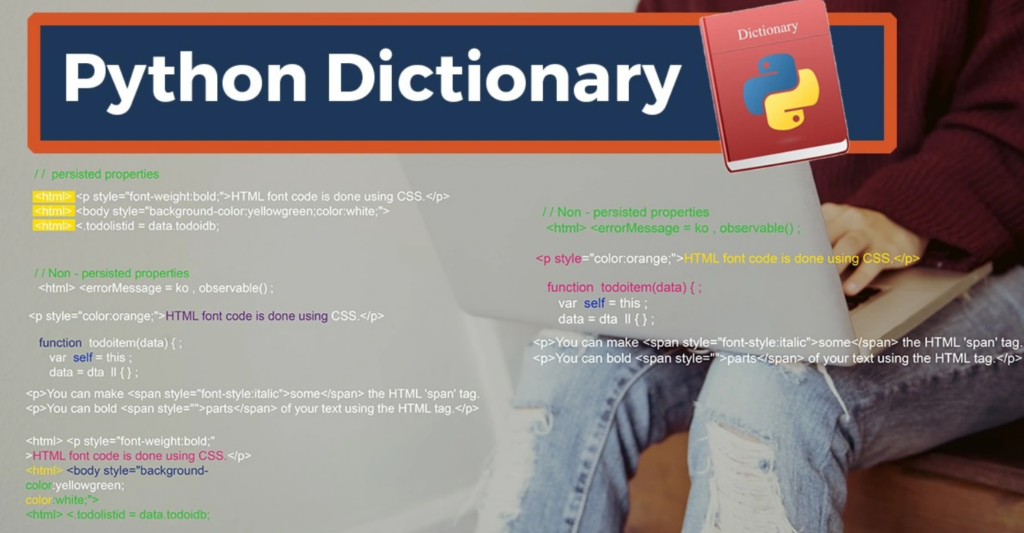
1. Understanding Python Dictionaries
A dictionary in Python is an unordered collection of data in a key/value pair format. Each key in a dictionary is unique and associated with a specific value. Dictionaries are flexible and can hold a wide range of data types as values, including numbers, strings, lists, and even other dictionaries. The ability to quickly look up values by their associated keys makes dictionaries a crucial data structure for many Python programs.
2. Creating and Initializing Python Dictionaries
Before we explore the art of adding key/value pairs, let’s start by understanding how to create and initialize dictionaries in Python.
You can create an empty dictionary using curly braces {}
my_dict = {}
Or, you can initialize a dictionary with key/value pairs:
student = {
“name”: “John”,
“age”: 20,
“grade”: “A”
}
3. Adding Key/Value Pairs
Adding key/value pairs to a dictionary is a straightforward process. You use the following syntax:
my_dict[key] = value
For example
# Creating an empty dictionary
my_dict = {}
#Adding key/value pairs
my_dict[“name”] = “Alice”
my_dict[“age”] = 25
print(my_dict) # Output: {‘name’: ‘Alice’, ‘age’: 25}
You can also add multiple key/value pairs at once:
# Adding multiple key/value pairs using update()
my_dict.update({“city”: “New York”, “country”: “USA”})
print(my_dict) # Output: {‘name’: ‘Alice’, ‘age’: 25, ‘city’: ‘New York’, ‘country’: ‘USA’}
4. Overwriting Values
If you add a key that already exists in the dictionary, the new value will overwrite the previous one:
my_dict = {“name”: “Alice”, “age”: 25}
# Overwriting a value
my_dict[“age”] = 26
print(my_dict) # Output: {‘name’: ‘Alice’, ‘age’: 26}
5. Using the update()
Method
The update()
method is a powerful tool for adding multiple key/value pairs to a dictionary. It takes a dictionary as an argument and adds its key/value pairs to the existing dictionary. If a key already exists, the method will update the value.
# Creating a dictionary
person = {“name”: “Alice”, “age”: 25}
# Using the update() method
person.update({“age”: 26, “city”: “New York”})
print(person) # Output: {‘name’: ‘Alice’, ‘age’: 26, ‘city’: ‘New York’}
The update()
method can also be used to add new key/value pairs:
# Creating a dictionary
person = {“name”: “Alice”, “age”: 25}
# Adding new key/value pairs using update()
person.update({“city”: “New York”, “country”: “USA”})
print(person) # Output: {‘name’: ‘Alice’, ‘age’: 25, ‘city’: ‘New York’, ‘country’: ‘USA’}
6. Adding Nested Dictionaries
Dictionaries in Python can also contain other dictionaries as values, creating nested data structures. This is especially useful for representing complex data. Here’s how you can add nested dictionaries:
# Creating a nested dictionary
employee = {
“name”: “John”,
“contact”: {
“email”: “john@example.com”,
“phone”: “123-456-7890”
}
}
7. Dictionary Comprehensions
Dictionary comprehensions are a concise way to create dictionaries in Python. They allow you to create dictionaries in a single line of code by specifying the key/value pairs you want to include.
Here’s an example of a dictionary comprehension that squares the values of a given dictionary:
original_dict = {“a”: 2, “b”: 3, “c”: 4}
squared_dict = {key: value ** 2 for key, value in original_dict.items()}
print(squared_dict) # Output: {‘a’: 4, ‘b’: 9, ‘c’: 16}
8. Tips and Best Practices
When working with dictionaries, consider the following tips and best practices:
- Check if a Key Exists: Before adding or modifying a key, it’s a good practice to check if the key already exists to avoid overwriting or raising errors. You can use the
in
operator or theget()
method to do this. - Use Descriptive Keys: Use descriptive and meaningful keys to make your code more readable and maintainable.
- Keep Keys Immutable: Dictionary keys must be immutable, which means they cannot be changed after they are created. Common examples of immutable types for keys are strings and tuples.
- Understand Key Order: In Python 3.7 and later, dictionaries maintain the order of key/value pairs as they were added. This means you can rely on the order in which items were added to the dictionary.
Conclusion
Python dictionaries are a fundamental data structure that provides a versatile and efficient way to store and manipulate data. Adding key/value pairs to dictionaries is a basic yet essential skill for any Python programmer. Whether you’re building complex data structures, managing configuration settings, or simply organizing data, dictionaries play a crucial role in your Python journey.
In this blog, we’ve delved into the art of adding key/value pairs to dictionaries, and we’ve also uncovered some powerful techniques and best practices. With this knowledge at your disposal, you can harness the full potential of Python’s dictionary capabilities, enabling you to work with data more effectively and efficiently in your Python programs.
Leave a Reply